Deploying Smart Contracts on Optimism: Best Practices for Developers
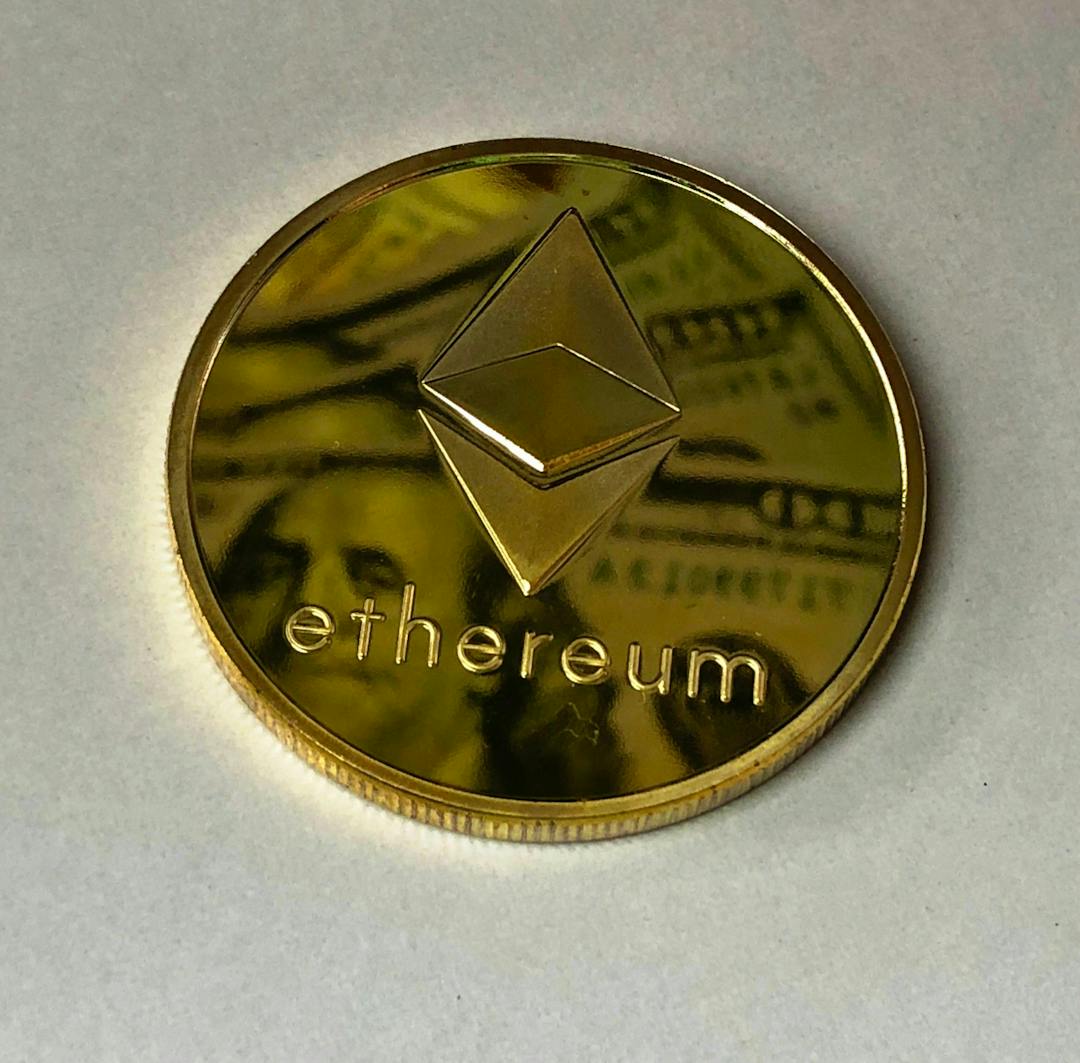
The landscape of decentralised finance (DeFi) and blockchain applications is evolving rapidly, with scalability and efficiency becoming increasingly crucial. Ethereum, while being the backbone of many decentralised applications (dApps), faces challenges with network congestion and high gas fees. Enter Optimism, a Layer 2 scaling solution designed to enhance Ethereum's capabilities by offering faster transactions and lower fees without compromising on security.
For developers looking to deploy smart contracts efficiently, Optimism presents a compelling platform. This blog delves into the best practices for deploying smart contracts on Optimism, ensuring you make the most of this innovative technology.
Understanding Optimism
What Is Optimism?
Optimism is a Layer 2 scaling solution for Ethereum that utilises Optimistic Rollups to increase transaction throughput and reduce fees. By processing transactions off-chain and only posting minimal data to the Ethereum mainnet, Optimism significantly alleviates congestion on the network.
How Does It Work?
Optimism operates on the principle of optimistic execution. Transactions are assumed to be valid and are batched together off-chain. These batches are then submitted to Ethereum with a proof. If any fraudulent activity is detected, a fraud-proof mechanism allows for disputes to be resolved on-chain, maintaining the integrity and security of the network.
Benefits of Using Optimism
Scalability: Increased transaction throughput allows for more complex and interactive dApps.
Lower Gas Fees: Significantly reduced fees make microtransactions and frequent interactions economically viable.
EVM Compatibility: Optimism is designed to be compatible with the Ethereum Virtual Machine (EVM), enabling developers to port existing smart contracts with minimal changes.
Preparing for Deployment
Setting Up Your Development Environment
Before deploying on Optimism, ensure your development environment is correctly configured.
- Node.js and NPM: Required for most Ethereum development tools.
- Solidity Compiler: Ensure you have the appropriate version of the Solidity compiler (solc).
- Ethereum Development Frameworks: Tools like Hardhat or Truffle are essential for compiling, testing, and deploying smart contracts.
- Optimism SDK: Provides utilities and tools specific to Optimism.
Network Configuration
Optimism has its own networks for testing and deployment:
- Optimism Goerli Testnet: Use this network for testing your contracts before mainnet deployment.
- Optimism Mainnet: For live deployment, connect to the Optimism mainnet.
Best Practices for Deploying Smart Contracts on Optimism
1. Ensure Solidity Compiler Compatibility
While Optimism strives for EVM equivalence, slight differences may exist.
- Compiler Version: Use a Solidity compiler version compatible with your smart contracts and Optimism's EVM.
- Language Features: Stick to Solidity language features that are supported on Optimism to avoid compilation or runtime errors.
2. Optimise Gas Usage
Even though gas fees are lower on Optimism, optimising gas consumption is still beneficial.
- Efficient Coding: Write clean and efficient code to reduce unnecessary computations.
- Use of Libraries: Utilise well-audited libraries that are optimised for gas efficiency.
- Batch Operations: Combine multiple operations into a single transaction where possible.
3. Correct Network Configuration
Misconfiguration can lead to failed deployments or lost funds.
- RPC Endpoints: Use the correct Remote Procedure Call (RPC) endpoints for Optimism networks.
- Network IDs: Ensure that your network configurations in development tools match those of Optimism.
- Deployment Scripts: Update deployment scripts to target Optimism networks specifically.
4. Thorough Testing
Testing is crucial to ensure your contracts function as intended on Optimism.
- Testnets: Deploy and test your contracts on the Optimism Goerli testnet.
- Unit Tests: Write comprehensive unit tests using frameworks like Mocha or Chai.
- Integration Tests: Test interactions between multiple contracts and with external systems.
5. Security Considerations
Security remains paramount when deploying smart contracts.
- Code Audits: Have your contracts audited by reputable security firms.
- Upgradable Contracts: Consider the use of proxy patterns for contract upgrades, but be cautious of the complexities they introduce.
- External Calls: Be wary of external contract calls, as they can introduce reentrancy vulnerabilities.
6. Leverage Optimism's EVM Equivalence
Optimism aims for full compatibility with Ethereum's EVM.
- Porting Contracts: Existing Ethereum contracts can often be deployed on Optimism with little to no modification.
- Tooling Compatibility: Use familiar Ethereum development tools, as many support Optimism out of the box.
- Community Resources: Engage with Optimism's developer community for support and shared knowledge.
7. Stay Updated with Optimism Developments
The Optimism ecosystem is rapidly evolving.
- Documentation: Regularly check Optimism's official documentation for updates.
- Community Channels: Join forums, Discord servers, and follow official social media channels.
- Software Updates: Keep your development tools and dependencies up to date to benefit from the latest features and security patches.
Common Pitfalls to Avoid
Misconfigured Network Settings
Incorrect RPC URLs, network IDs, or gas settings can lead to failed transactions or deployments on the wrong network.
- Double-Check Configurations: Always verify your network settings before deploying.
- Environment Variables: Use environment variables to manage configurations for different networks.
Ignoring Optimism's Nuances
While Optimism strives for EVM equivalence, there are subtle differences.
- Transaction Finality: Understand that transaction confirmations might work differently.
- Supported Features: Some Ethereum features may not be fully supported or might behave differently.
Overlooking Gas Price Settings
Gas prices on Optimism are lower but still exist.
- Dynamic Gas Pricing: Use tools that can adjust gas prices dynamically based on network conditions.
- Default Values: Do not rely on Ethereum mainnet gas price defaults; adjust them for Optimism.
Step-by-Step Deployment Guide
1. Install Dependencies
Ensure you have all necessary tools installed:
// bash npm install --save-dev hardhat @nomiclabs/hardhat-ethers ethers npm install --save-dev @eth-optimism/plugins/hardhat
2. Configure Hardhat for Optimism
Update your hardhat.config.js:
// javascript require('@eth-optimism/plugins/hardhat/ethers'); module.exports = { networks: { optimism: { url: 'https://mainnet.optimism.io', accounts: [process.env.PRIVATE_KEY], chainId: 10, }, optimismGoerli: { url: 'https://goerli.optimism.io', accounts: [process.env.PRIVATE_KEY], chainId: 420, }, }, solidity: '0.8.17', };
3. Write and Compile Your Smart Contract
Create a simple contract, e.g., Greeter.sol:
solidity
// SPDX-License-Identifier: MIT pragma solidity ^0.8.17; contract Greeter { string greeting; constructor(string memory _greeting) { greeting = _greeting; } function greet() public view returns (string memory) { return greeting; } }
Compile the contract:
// bash npx hardhat compile
4. Deploy to Optimism Testnet
Create a deployment script, deploy.js:
// javascript async function main() { const Greeter = await ethers.getContractFactory("Greeter"); const greeter = await Greeter.deploy("Hello, Optimism!"); await greeter.deployed(); console.log("Greeter deployed to:", greeter.address); } main() .then(() => process.exit(0)) .catch((error) => { console.error(error); process.exit(1); });
Run the deployment:
// bash npx hardhat run scripts/deploy.js --network optimismGoerli
5. Verify Deployment
Check Explorer: Use the Optimism block explorer to verify your contract's deployment.
Interact with Contract: Use tools like Etherscan or MyEtherWallet to interact with your contract on the testnet.
Conclusion
Deploying smart contracts on Optimism offers numerous benefits, from reduced gas fees to enhanced scalability. By following best practices and being mindful of Optimism's unique features, developers can efficiently deploy and manage their smart contracts on this Layer 2 solution.
As the blockchain ecosystem continues to grow, leveraging platforms like Optimism will be instrumental in building scalable and user-friendly decentralised applications. By staying informed and adopting these best practices, developers can play a pivotal role in advancing the DeFi landscape.
At Mighty Labs, we are dedicated to empowering developers with the knowledge and tools needed to succeed in the ever-evolving world of blockchain technology. Contact us today to learn how we can assist you in deploying high-performance smart contracts on Optimism and other leading platforms.